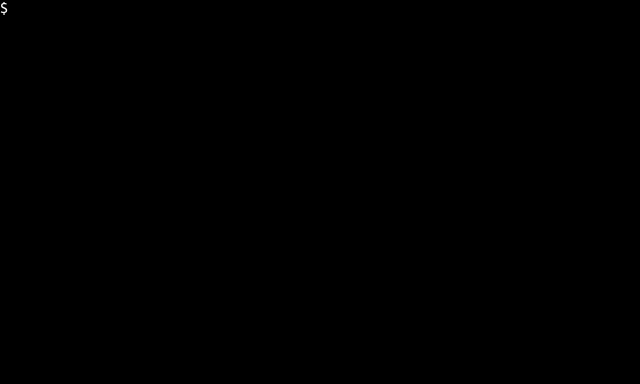
import org.scalameter.api._
object RangeBenchmark extends Bench.ForkedTime {
val ranges = for {
size <- Gen.range("size")(300000, 1500000, 300000)
} yield 0 until size
measure method "map" in {
using(ranges) curve("Range") in {
_.map(_ + 1)
}
}
}
ScalaMeter is a microbenchmarking and performance regression testing framework for the JVM platform that allows expressing performance tests in a way which is both simple and concise.
It can be used both from Scala and Java.
- write performance tests in a DSL similar to ScalaTest and ScalaCheck
- specify test input data
- specify how test results are collected
- organize performance tests hierarchically
After writing a performance test, you run it. You can configure how the test is executed depending on whether you need to compare several alternatives, check for performance regressions or just obtain the running time of a simple microbenchmark.
- decide how and where the tests are executed
- automatically eliminate noise due to JIT compilation, garbage collection or undesired heap allocation patterns
- customize test results reporting
- save performance test results for later analysis
$ scala -cp scalameter_2.10-0.1.jar:. RangeBenchmark
::Benchmark map::
jvm-name: Java HotSpot(TM) 64-Bit Server VM
jvm-vendor: Oracle Corporation
jvm-version: 23.0-b16
os-arch: amd64
os-name: Mac OS X
Parameters(size -> 300000): 2.0
Parameters(size -> 600000): 4.0
Parameters(size -> 900000): 6.0
Parameters(size -> 1200000): 8.0
Parameters(size -> 1500000): 11.0
class MapBenchmarks extends JBench.ForkedPreciseTime {
@gen("hashtries")
@benchmark("maps.apply")
@curve("hash-trie")
def hashTrieApply(xs: HashMap[Int, Int]): Long = {
var i = 0, sum = 0L
while (i < xs.size) {
sum += xs.apply(i)
i += 1
}
sum
}
}
ScalaMeter tests can also be defined using an annotation-based declaration style, where each benchmark is an annotated method. This style is usable from both Java and Scala, and is more friendly towards users familiar with JUnit and similar testing frameworks.
- define generators for your input data
- set per-benchmark configuration options
- organized tests into separate plots (i.e. scopes) and curves within those plots
Features
- quick and easy setup for simple microbenchmarks
- multiple configurable JVM microbenchmarking methodologies
- concise DSL for performance tests and input data generation
- configurable performance test statistical analysis and automated regression detection
See the Getting Started section for a more detailed explanation.